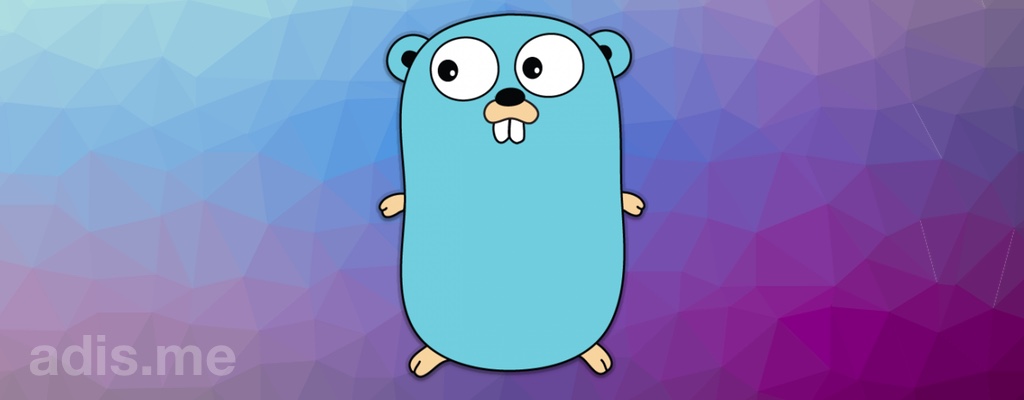
Looking for a Golang rocket launch project? Check out these handpicked components. Each component is perfectly designed for its job.
- Router - http://www.gorillatoolkit.org/pkg/mux
- Middleware - https://github.com/urfave/negroni (alt: http://www.gorillatoolkit.org/pkg/handlers)
- Logging - https://github.com/sirupsen/logrus
- Database ORM - https://github.com/jinzhu/gorm
- Sessions - http://www.gorillatoolkit.org/pkg/Sessions
- Schema bindings - http://www.gorillatoolkit.org/pkg/Schema
- Configuration - https://github.com/BurntSushi/toml
When you glue these components into one project you will get a rocket powered Go project. Oh boy, this project is already for deployment. And all your customers would be happy.
In your root folder create a main.go
file that looks like the following:
package main
import (
"log"
"net/http"
"time"
"github.com/gorilla/mux"
"github.com/urfave/negroni"
)
func main() {
// Instantiate new mux router
r := mux.NewRouter()
// Instantiate middleware provider
n := negroni.Classic()
n.UseHandler(r)
// Register application routes
r.HandleFunc("/", new(handlers.HomeHandler).Index)
// Prepare server to spin up
s := &http.Server{
Addr: "127.0.0.1:8000",
// link middleware with router
Handler: n,
// default time outs
WriteTimeout: 15 * time.Second,
ReadTimeout: 15 * time.Second,
}
// Listen and serve baby
log.Fatal(s.ListenAndServe())
}
In the above file I assume you have a folder called handlers/http/
. In this folder at least a HomeHandler
is present with the following content:
package handlers
import (
"fmt"
"net/http"
)
// HomeHandler represents basic routes
type HomeHandler struct{}
// Index will show the homepage
func (h HomeHandler) Index(w http.ResponseWriter, r *http.Request) {
fmt.Fprint(w, "Hi there, I am ready!")
}
This post will be updated shortly.
golang